Calculated Fields in Surveys
Learn how to configure calculated fields in surveys using JavaScript for advanced analysis and manipulation of data collected from survey responses.
Introduction
Calculated fields in surveys enable you to perform operations on the data collected in a survey, providing a more detailed and nuanced analysis of the responses. Configuring calculated fields involves the use of JavaScript to define the logic behind the calculations. The calculations can range from simple mathematical operations, such as addition and subtraction, to more complex functions involving conditionals and loops.
JavaScript code can reference other fields in the survey and perform programatic calculations. The result is then stored in the calculated field as an integer.
You can configure the logic used in calculated fields to suit your needs. For example, you can use conditional logic to assign different scores based on specific answers, or use loops to calculate a total score when multiple answers are selected. The flexibility of JavaScript allows for a wide range of scenarios, making calculated fields a powerful tool for data analysis in surveys.
Note
In calculated fields, ECMAScript 6 (also known as ES6 or ECMAScript 2015) is supported.
Configuring Calculated Fields
To configure a calculated field when creating a survey, drag the Calculated field question from the left-side panel to the survey body, then add JavaScript code to the field.
You can add multiple calculated fields to a survey. Calculated fields are not shown to survey respondents, but they appear in the survey preview.
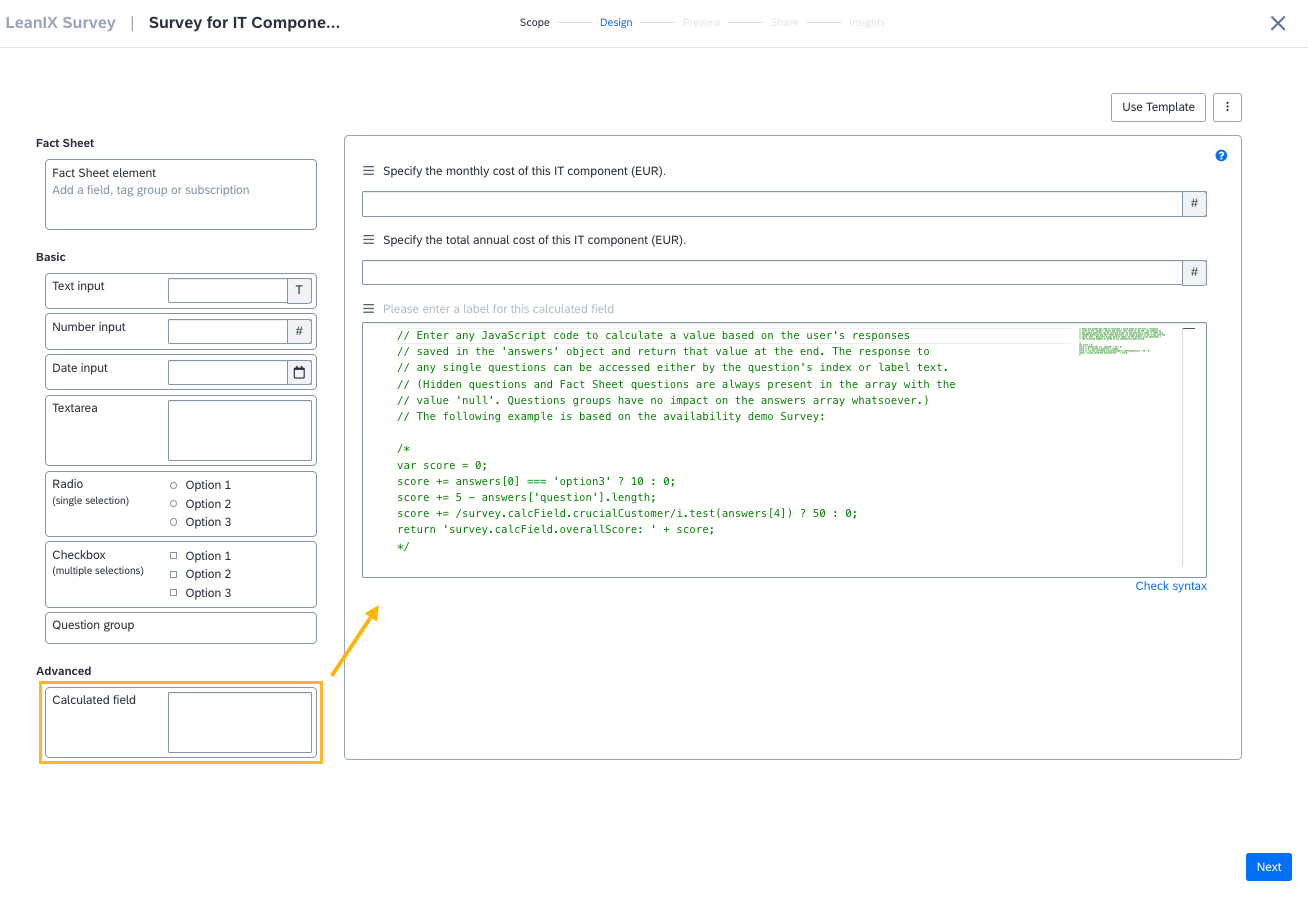
Adding a Calculated Field to a Survey
Processing Answers in Calculated Fields
In calculated fields, the answers
array is a built-in variable that holds the responses to the survey questions. Each element in the answers
array corresponds to a question in the survey, in the order that the questions are defined.
Here's how you can use the answers
array when configuring calculated fields:
- Referencing answers: Determine the order of the question whose answer you want to use in the calculation. Use
answers[index]
in your JavaScript code to reference the answer to a specific question. Replaceindex
with the order of the question. Remember that the order is zero-based, so the first question's answer is at index 0 (answers[0]
), the second question's answer is at index 1 (answers[1]
), and so on. - Using answers in calculations: You can use the values in the
answers
array in your calculations. For example, if you're calculating the sum of two numeric responses, you can useNumber(answers[0]) + Number(answers[1])
. - Handling different answer types: The type of the value in the
answers
array depends on the question type. For single-select or text input questions, the value is a string. For multiple-select questions, the value is an array of strings. For numeric questions, the value is a string that can be converted to a number. - Using conditional logic: You can use conditional logic to check for specific answers. For example,
answers[0] === 'Yes'
checks if the answer to the first question is “Yes”.
The result of the calculation should be returned at the end of the JavaScript code, for example, return sum;
. This is the value that is stored in the calculated field.
Writing Calculated Fields to Fact Sheets
Calculated fields are not automatically written to fact sheets. You can update fact sheets with calculated field values by implementing a code-based automation using APIs.
For example, you can set up a code-based automation to update custom fields on a fact sheet with calculated risk scores and map them to user-friendly readable values. To learn how to implement this automation, please see Calculating Risk Scores and Updating a Fact Sheet Based on Survey Responses in our developer documentation.
Examples
In this section, you can find examples of JavaScript code for some typical use cases.
Number Input Questions: Calculating the Sum of Answers
When using number input questions in surveys, you can configure calculated fields to perform simple mathematical operations, such as calculating the sum or average of submitted values. For example, you can create a survey that asks for the monthly cost and annual maintenance cost of a specific IT component and then use a calculated field to compute the total cost of the component over a year.
Questions:
- Specify the monthly cost of this IT component.
- Specify the annual maintenance cost of this IT component.
Here's how you can set up a calculated field for this scenario:
// Add the monthly cost (multiplied by 12 for annual cost) to the total cost
var monthlyCost = Number(answers[0]) * 12;
// Add the annual maintenance cost to the total cost
var annualMaintenanceCost = Number(answers[1]);
// Total cost is monthlyCost + annualMaintenanceCost
totalCost = monthlyCost + annualMaintenanceCost;
// Return the total annual cost of the IT component in the calculated field
return totalCost;
In this code, answers[0]
refers to the answer to the first question in the survey (the monthly cost), and answers[1]
refers to the answer to the second question (the annual maintenance cost). The Number()
function is used to ensure that the answers are treated as numbers, not strings. The return totalCost;
statement at the end ensures that the total annual cost is returned in the calculated field.
Single-Select Questions: Assigning Scores to Answers
When using single-select (radio) questions in surveys, you can configure a calculated field to assign a score to each answer, which enables you to rate or rank responses. You can also use the selected answer in conditional logic to perform different calculations based on the response. For example, you can calculate a different score or perform a different operation depending on which option was selected.
The question in this example is a single-select (radio) question with three answer options. The objective here is to assign a score to the submitted answer.
Question: Assess the compliance of this technology with our organization's security standards.
Answer options and scores:
- Fully compliant: 1
- Partially compliant: 2
- Not compliant: 3
Here's how you can set up a calculated field for this scenario:
// Initialize a score mapping
const scoreMapping = {
'Fully compliant': 1,
'Partially compliant': 2,
'Not compliant': 3
};
// Calculate the score using the mapping, defaulting to 0 for unexpected answers
let score = scoreMapping[answers[0]] || 0;
// Return the score in the calculated field
return score;
In this code, answers[0]
refers to the answer given to the first question in the survey. Because it’s a single-select question, only one answer is possible. The return score;
statement at the end ensures that the score is returned in the calculated field. You can use this score to assess risks associated with a specific technology.
Multiple-Select Questions: Assigning Scores to Answers
When using multiple-select (checkbox) questions in surveys, you can configure a calculated field to assign scores to each selected option, which allows for a more nuanced understanding of responses. This approach facilitates the aggregation of data, making it easier to identify patterns, trends, and correlations within the responses.
The question in this example is a multiple-select (checkbox) question with six answer options. The objective here is to assign a score to each selected answer option and then calculate the total score.
Question: Which potential technological risks is the organization prepared to handle? Select all that apply.
Answer options and scores:
- Cybersecurity breaches: 3
- Data privacy issues: 5
- Technology failure: 7
- Integration risks: 3
- Technology obsolescence: 5
- AI and automation risks: 3
Here's how you can set up a calculated field for this scenario:
// Map each answer option to its corresponding score
const answerScore = {
'Cybersecurity breaches': 3,
'Data privacy issues': 5,
'Technology failure': 7,
'Integration risks': 3,
'Technology obsolescence': 5,
'AI and automation risks': 3
};
// Initialize a variable to hold the total score
let totalScore = 0;
// Get the answer to the first question
const answer = answers[0];
// Check if multiple options were selected (the answer would be an array)
if (Array.isArray(answer)) {
// If multiple options were selected, go through each one
// Add the score corresponding to each selected option to the total score
totalScore = answer.reduce((score, ans) => score + (answerScore[ans] || 0), 0);
}
// Return the overall sum of scores in the calculated field
return totalScore;
In this code, answers[0]
refers to the answer to the first question in the survey. The Array.isArray(answer)
check is used to ensure that the answer is an array. If multiple options are selected for this question, the code loops through each selected option and adds the corresponding score to totalScore
.
The return totalScore;
statement at the end ensures that the calculated field will hold the total score based on the selected options. This provides a quick and effective way to quantify an organization's preparedness to handle various technological risks directly within your survey.
Text Input Questions: Assigning Scores If a Specific Text Is Found
When using text input questions in surveys, you can configure a calculated field to assign scores based on whether a specific text or keyword is found in the response. This is especially useful when you want to quantify the frequency or importance of certain themes or issues mentioned by respondents.
Question: What are the top three challenges you face in managing the application?
A respondent might answer: "Slow response times or other performance-related issues."
You can assign a score based on whether specific keywords (for example, "performance" or "slow") appear in the response. Here's how you can set up a calculated field for this scenario:
// Assign a variable for the answer of the first question
const sentence = answers[0];
// Initialize a score and assign a score based on a specific text/keyword in the sentence
let score = 0;
if (sentence.includes("performance")) {
score = 10;
} else if (sentence.includes("slow")) {
score = 5;
}
// Return the score in the calculated field
return score;
In this code, answers[0]
refers to the response to the first question in the survey. The includes()
method is used to check if the response contains the keywords "performance" or "slow". If the keyword "performance" is found in the response, a score of 10 is assigned. If the keyword "slow" is found, a score of 5 is assigned. If neither keyword is found, a score of 0 is assigned.
The return score;
statement at the end ensures that the score is returned in the calculated field. This provides a simple and effective way to quantify and analyze open-ended text responses directly within the survey.
Assigning Weights to Questions
When using calculated fields, you can assign weights to different questions, which allows you to emphasize certain questions over others in your analysis. This is particularly useful when some questions in your survey are more important than others. Weights can be distributed equally or unequally amongst the questions based on your specific needs.
The survey in this example has four questions, where each question has a different weight based on its importance. The second question is considered more important and thus assigned a larger weight. All questions are of the single-select (radio) type.
Question | Weight | Answer Options and Scores |
---|---|---|
Assess the compliance of this technology with our organization's security standards. | 0.25 | - Fully compliant:1 - Partially compliant: 2 - Not compliant: 3 |
Rate the level of support provided by the vendor for this technology. | 0.40 | - Excellent support: 1 - Adequate support: 2 - Poor support: 3 |
Assess the sensitivity of the data handled by this technology. | 0.10 | - Low sensitivity: 1 - Medium sensitivity: 2 - High sensitivity: 3 |
Assess the potential impact on operations if this technology were to fail. | 0.25 | - Low impact: 1 - Medium impact: 2 - High impact: 3 |
Here's how you can set up a calculated field for this scenario:
// Define the weight for each question, the total weight is 1 (100%)
const wb1 = 0.25; // weight for first question
const wb2 = 0.40; // weight for second question
const wb3 = 0.10; // weight for third question
const wb4 = 0.25; // weight for fourth question
// Initialize a variable for the score of each question
var score1 = 0; // for first question
var score2 = 0; // for second question
var score3 = 0; // for third question
var score4 = 0; // for fourth question
// Initialize a variable for the total score
var totalScore = 0;
// Assign a value to each answer of the first question
score1 += answers[0] === 'Fully compliant' ? 1 : 0;
score1 += answers[0] === 'Partially compliant' ? 2 : 0;
score1 += answers[0] === 'Not compliant' ? 3 : 0;
// Assign a value to each answer of the second question
score2 += answers[1] === 'Excellent support' ? 1 : 0;
score2 += answers[1] === 'Adequate support' ? 2 : 0;
score2 += answers[1] === 'Poor support' ? 3 : 0;
// Assign a value to each answer of the third question
score3 += answers[2] === 'Low sensitivity' ? 1 : 0;
score3 += answers[2] === 'Medium sensitivity' ? 2 : 0;
score3 += answers[2] === 'High sensitivity' ? 3 : 0;
// Assign a value to each answer of the fourth question
score4 += answers[3] === 'Low impact' ? 1 : 0;
score4 += answers[3] === 'Medium impact' ? 2 : 0;
score4 += answers[3] === 'High impact' ? 3 : 0;
// Calculate the total score by multiplying each question score by its corresponding weight and summing up all scores
totalScore = score1*wb1 + score2*wb2 + score3*wb3 + score4*wb4;
// Return the total weighted score in the calculated field
return totalScore;
In this code, each question has a different weight (wb1
, wb2
, and so on), and a score is calculated for each question based on the answers. The total score is then calculated by multiplying each question score by its corresponding weight and summing up all the scores. This provides a weighted total score that takes into account the importance of each question.
Updated about 1 month ago